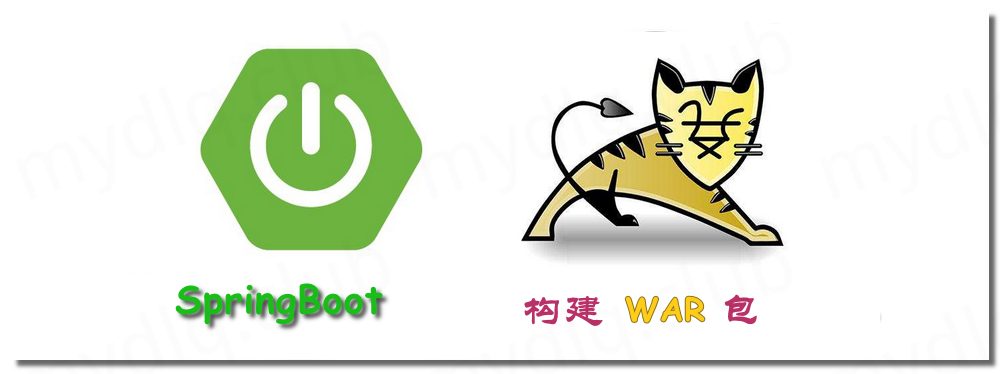
SpringBoot 应用构建 WAR 包
文章目录
!版权声明:本博客内容均为原创,每篇博文作为知识积累,写博不易,转载请注明出处。
系统环境:
- JDK 版本:1.8
- SpringBoot 版本:2.2.4.RELEASE
参考地址:
- 博文示例项目 Github 地址:https://github.com/my-dlq/blog-example/tree/master/springboot/springboot-war-example
一、简介
SpringBoot 中默认内置 Tomcat 容器,一般是构建 Jar 包方式,但是有时候需要使用外置的 Tomcat 来部署应用,这时候需要将应用打包成 War 包。
SpringBoot 打包 War 方式也很简单,下面将介绍下如何将 SpringBoot 应用打包成 War 包。
二、SpringBoot 构建 WAR 包
1、pom 中指定以 War 方式 打包
1<packaging>war</packaging>
2、SpringBoot Web 排除 Tomcat 依赖
SpringBoot Web 排除 Tomcat 依赖,并且单独引入 Tomcat 依赖,设置 Tomcat 依赖只应用于 编译
、测试
、运行
阶段,但是 打包
阶段将会移除该依赖。
1<dependencies>
2 <!--SpringBoot Web 依赖,并且排除 SpringBoot Web 中的 Tomcat 依赖-->
3 <dependency>
4 <groupId>org.springframework.boot</groupId>
5 <artifactId>spring-boot-starter-web</artifactId>
6 <exclusions>
7 <exclusion>
8 <groupId>org.springframework.boot</groupId>
9 <artifactId>spring-boot-starter-tomcat</artifactId>
10 </exclusion>
11 </exclusions>
12 </dependency>
13 <!--添加 Tomcat 依赖,并且设置只在编译,测试,运行等时候应用 Tomcat 依赖,但是打包阶段将会移除该依赖-->
14 <dependency>
15 <groupId>org.springframework.boot</groupId>
16 <artifactId>spring-boot-starter-tomcat</artifactId>
17 <scope>provided</scope>
18 </dependency>
19</dependencies>
3、指定构建的 WAR 包名称
1<build>
2 <!--定义 War 包名称-->
3 <finalName>WAR包名称</finalName>
4 <plugins>
5 <plugin>
6 <groupId>org.springframework.boot</groupId>
7 <artifactId>spring-boot-maven-plugin</artifactId>
8 </plugin>
9 </plugins>
10</build>
4、启动类继承 SpringBootServletInitializer 依赖
启动类中继承 SpringBootServletInitializer 类,并且配置实现 configure 方法:
1@SpringBootApplication
2public class Application extends SpringBootServletInitializer {
3
4 @Override
5 protected SpringApplicationBuilder configure(SpringApplicationBuilder builder) {
6 return builder.sources(Application.class);
7 }
8
9 public static void main(String[] args) {
10 SpringApplication.run(Application.class, args);
11 }
12
13}
三、示例应用
1、Pom.xml 进行配置
1<?xml version="1.0" encoding="UTF-8"?>
2<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
3 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
4 <modelVersion>4.0.0</modelVersion>
5
6 <parent>
7 <groupId>org.springframework.boot</groupId>
8 <artifactId>spring-boot-starter-parent</artifactId>
9 <version>2.2.4.RELEASE</version>
10 </parent>
11
12 <groupId>mydlq.club</groupId>
13 <artifactId>springboot-war-example</artifactId>
14 <version>0.0.1</version>
15 <name>springboot-war-example</name>
16 <description>springboot war example</description>
17
18 <!--指定以 War 方式打包-->
19 <packaging>war</packaging>
20
21 <properties>
22 <java.version>1.8</java.version>
23 </properties>
24
25 <dependencies>
26 <!--SpringBoot Web 依赖,并且排除 SpringBoot Web 中的 Tomcat 依赖-->
27 <dependency>
28 <groupId>org.springframework.boot</groupId>
29 <artifactId>spring-boot-starter-web</artifactId>
30 <exclusions>
31 <exclusion>
32 <groupId>org.springframework.boot</groupId>
33 <artifactId>spring-boot-starter-tomcat</artifactId>
34 </exclusion>
35 </exclusions>
36 </dependency>
37 <!--添加 Tomcat 依赖,并且设置只在编译,测试,运行等时候应用 Tomcat 依赖,但是打包阶段将会移除该依赖-->
38 <dependency>
39 <groupId>org.springframework.boot</groupId>
40 <artifactId>spring-boot-starter-tomcat</artifactId>
41 <scope>provided</scope>
42 </dependency>
43 </dependencies>
44
45 <build>
46 <!--定义 War 包名称-->
47 <finalName>helloworld</finalName>
48 <plugins>
49 <plugin>
50 <groupId>org.springframework.boot</groupId>
51 <artifactId>spring-boot-maven-plugin</artifactId>
52 </plugin>
53 </plugins>
54 </build>
55
56</project>
2、创建示例接口 Controller 类
1import org.springframework.web.bind.annotation.GetMapping;
2import org.springframework.web.bind.annotation.RestController;
3
4@RestController
5public class TestController {
6
7 @GetMapping("/hello")
8 public String hello1() {
9 return "hello world!";
10 }
11
12}
3、SpringBoot 启动类配置
1import org.springframework.boot.SpringApplication;
2import org.springframework.boot.autoconfigure.SpringBootApplication;
3import org.springframework.boot.builder.SpringApplicationBuilder;
4import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
5
6@SpringBootApplication
7public class Application extends SpringBootServletInitializer {
8
9 @Override
10 protected SpringApplicationBuilder configure(SpringApplicationBuilder builder) {
11 return builder.sources(Application.class);
12 }
13
14 public static void main(String[] args) {
15 SpringApplication.run(Application.class, args);
16 }
17
18}
四、进行测试
1、将项目构建 WAR 包
使用 Maven 执行打包
1$ mvn clean package
2、下载并配置 Tomcat
1#下载 Tomcat
2$ wget http://mirrors.tuna.tsinghua.edu.cn/apache/tomcat/tomcat-9/v9.0.30/bin/apache-tomcat-9.0.30.tar.gz
3
4#解压 Tomcat
5$ tar -zxf apache-tomcat-9.0.30.tar.gz
6
7#重命名
8$ mv ./apache-tomcat-9.0.30 ./tomcat
3、将 WAR 包放置到 Tomcat 进行测试
1$ cp /apps/helloworld.war /usr/local/tomcat/bin/helloworld.war
4、启动 Tomcat
1$ /usr/local/tomcat/bin/startup.sh
5、访问服务
访问示例应用的服务地址,可以看到 hello world!
字样。
1$ curl http://localhost:8080/helloworld/hello
2
3hello world!
6、关闭 Tomcat
测试完成,关闭 Tomcat:
1$ /usr/local/tomcat/bin/shutdown.sh
---END---

!版权声明:本博客内容均为原创,每篇博文作为知识积累,写博不易,转载请注明出处。